Сортируемая таблица на ReactJS
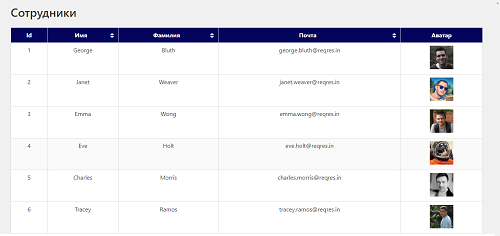
Доброго времени суток! Сегодня я покажу Вам как можно создать сортируемую таблицу с помощью ReactJS. Сборка происходит с помощью ParcelJS.
В корне проекта следующий package.json:
{
"name": "staff-app",
"version": "0.1.0",
"scripts": {
"dev": "rm -rf ./.cache && rm -rf ./dist && npx parcel src/index.html",
"prod": "rm -rf ./.cache && rm -rf ./dist && npx parcel build src/index.html"
},
"dependencies": {
"bulma": "^0.9.0",
"react": "^16.13.1",
"react-dom": "^16.13.1"
},
"devDependencies": {
"parcel-bundler": "^1.12.4"
}
}
Папка src
D:.
│ index.html
│ main.js
│
└───components
│ App.css
│ App.js
│
└───SortableTable
sortable-table.css
SortableTable.js
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Сотрудники</title>
<!-- Font Awesome -->
<script defer src="https://kit.fontawesome.com/921224fb4e.js"></script>
</head>
<body>
<div id="root"></div>
<script src="./main.js"></script>
</body>
</html>
main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './components/App';
ReactDOM.render(
<App />,
document.getElementById('root')
);
./components/App.js
import React from 'react';
import './App.css';
import SortableTable from './SortableTable/SortableTable'
const App = () => {
return (
<div className="container is-fluid">
<h1 className="title mt-5">Сотрудники</h1>
<SortableTable firstName="Имя" lastName="Фамилия" email="Почта" avatar="Аватар" />
</div>
);
};
export default App;
./components/SortableTable/SortableTable.js
import React, { Component } from 'react';
import 'bulma/css/bulma.min';
import './sortable-table.css';
class SortableTable extends Component {
constructor(props) {
super(props);
this.state = { data: [] };
this.onSort = this.onSort.bind(this)
}
componentDidMount() {
fetch('https://reqres.in/api/users')
.then(function(response) {
return response.json();
})
.then(items => this.setState({ data: items.data }));
}
onSort(event, sortKey){
const data = this.state.data;
data.sort((a,b) => a[sortKey].localeCompare(b[sortKey]))
this.setState({data})
}
render() {
let newdata = this.state.data;
let { firstName, lastName, email, avatar } = this.props;
return (
<table className="table is-bordered is-hoverable is-fullwidth has-text-centered">
<thead>
<tr>
<th onClick={e => this.onSort(e, 'id')}>Id</th>
<th onClick={e => this.onSort(e, 'first_name')}>
{firstName}
<i className="fas fa-sort"></i>
</th>
<th onClick={e => this.onSort(e, 'last_name')}>
{lastName}
<i className="fas fa-sort"></i>
</th>
<th onClick={e => this.onSort(e, 'email')}>
{email}
<i className="fas fa-sort"></i>
</th>
<th>{avatar}</th>
</tr>
</thead>
<tbody>
{newdata.map(function(user) {
return (
<tr key={user.id}>
<td>{user.id}</td>
<td>{user.first_name}</td>
<td>{user.last_name}</td>
<td>{user.email}</td>
<td><img src={user.avatar} width={64}></img></td>
</tr>
);
})}
</tbody>
</table>
);
}
}
export default SortableTable;
./components/SortableTable/sortable-table.css
th {
cursor: pointer;
background-color: rgb(4, 4, 92);
color: white !important;
}
th i {
float: right;
margin-top: 5px;
}
В итоге должно получиться следующее:
При нажатии на стрелочки в заголовке таблицы данные сортируются.
-
-
Михаил Русаков
Комментарии (0):
Для добавления комментариев надо войти в систему.
Если Вы ещё не зарегистрированы на сайте, то сначала зарегистрируйтесь.