Счетчик обратного счета на JavaScript с возможностью рестарта
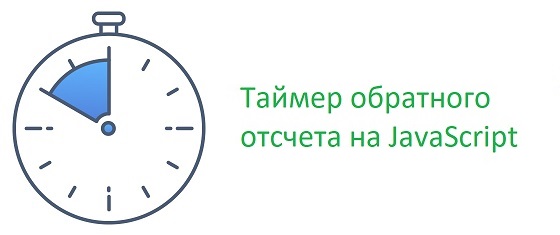
Скрипт счетчика:
function CountDownTimer(duration, granularity) {
this.time_format = this.getTimeFormat(duration);
this.duration = this.toSeconds(duration);
this.initialDuration = this.duration;
this.granularity = granularity || 1000;
this.running = false;
this.onStopCallback = undefined;
this.onRestartCallback = undefined;
this.tickFunctions = [];
this.timerHandle = undefined;
}
CountDownTimer.TIME_FORMAT_HHMMSS = "hh:mm:ss";
CountDownTimer.TIME_FORMAT_MMSS = "mm:ss";
CountDownTimer.prototype.getTimeFormat = function( timeStr )
{
let formats = [ /^\d{2}:\d{2}:\d{2}$/, /^\d{2}:\d{2}$/ ];
if( formats[0].test(timeStr) ) {
return CountDownTimer.TIME_FORMAT_HHMMSS;
}
if( formats[1].test(timeStr) ) {
return CountDownTimer.TIME_FORMAT_MMSS;
}
if( !timeStr ) return;
throw new Error('Unsupported time format!');
};
CountDownTimer.prototype.onTick = function (callback) {
if (typeof callback == 'function') {
this.tickFunctions.push(callback);
}
return this;
};
CountDownTimer.prototype.toSeconds = function (str) {
let timeParts = str.split(':');
let hours, minutes, seconds;
if ( this.time_format === CountDownTimer.TIME_FORMAT_MMSS ) {
[minutes, seconds] = timeParts;
return (+minutes) * 60 + (+seconds);
}
if ( this.time_format === CountDownTimer.TIME_FORMAT_HHMMSS ) {
[hours, minutes, seconds] = timeParts;
}
return (+hours) * 3600 + (+minutes) * 60 + (+seconds);
};
CountDownTimer.prototype.toTimeObject = function (seconds) {
//if (seconds <= 3599) {
if ( this.time_format === CountDownTimer.TIME_FORMAT_MMSS ) {
return {
minutes: (seconds / 60) | 0,
seconds: (seconds % 60) | 0
};
}
return {
hours: (seconds / 3600) | 0,
minutes: ((seconds / 60) | 0) % 60,
seconds: (seconds % 60) | 0
};
};
CountDownTimer.prototype.zeroPad = function (time) {
let pad = (value) => value < 10 ? '0' + value : value;
let _time = [];
for( let prop in time ) {
if( time.hasOwnProperty(prop) ) _time.push(pad(time[prop]));
}
return _time.join(':');
};
CountDownTimer.prototype.format = function (seconds) {
return this.zeroPad(this.toTimeObject(seconds));
};
CountDownTimer.prototype.updateDuration = function(duration) {
this.time_format = this.getTimeFormat(duration);
this.duration = this.toSeconds(duration);
this.initialDuration = this.duration;
return this;
};
CountDownTimer.prototype.start = function () {
// if timer is running simply return from it
if (this.running) {
return;
}
this.running = true;
let that = this, diff;
(function timer() {
diff = that.duration--;
if (diff >= 0) {
that.timerHandle = setTimeout(timer, that.granularity);
} else {
// if onRestart callback exists
// restart timer
if( that.onRestartCallback ) {
that.duration = that.initialDuration;
that.running = false;
clearTimeout(that.timerHandle);
that.timerHandle = setTimeout(timer, that.granularity);
that.onRestartCallback.call(that,that);
return;
}
diff = 0;
that.running = false;
clearTimeout(that.timerHandle);
if (that.onStopCallback) that.onStopCallback.call(null,that);
return;
}
that.tickFunctions.forEach(function (callback) {
callback.call(this, that);
}, that);
}());
};
CountDownTimer.prototype.onStop = function (callback) {
this.onStopCallback = callback;
return this;
};
CountDownTimer.prototype.onRestart = function(callback) {
this.onRestartCallback = callback;
return this;
};
CountDownTimer.prototype.stop = function() {
this.duration = -1;
this.running = false;
clearTimeout(this.timerHandle);
};
Используется так:
// div блок
let el = element.classList.contains("div");
// внутрений текст "00:00:00" или "00:00"
let timer = new CountDownTimer(el.innerText);
// на отсчет таймера
timer.onTick((time) => el.innerText = timer.format(time));
// рестарт таймер при окончании
// здесь можно выполнять любое действие
// необходимое вам
timer.onRestart((self) => {
alert("Время закончилось")
});
timer.start();
-
-
Михаил Русаков
Комментарии (0):
Для добавления комментариев надо войти в систему.
Если Вы ещё не зарегистрированы на сайте, то сначала зарегистрируйтесь.