Пишем приложение на React
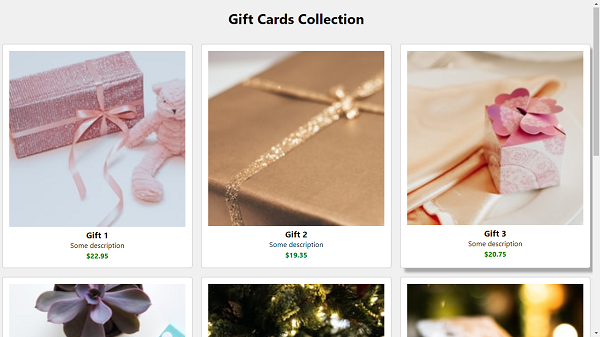
В данной статье я расскажу Вам как можно быстро создать приложение на ReactJS. Наше приложение будет отображать коллекцию подарочных карточек и будет иметь отзывчивый интерфейс.
Итак, начнем с установки самого набора инструментов для разработки на React. Здесь особенно ничего сложного нет, однако есть один существенный момент, если у Вы работаете на Windows, то могут возникнуть проблемы в установке из-за пробелов в путях к файлам. Поэтому, проследите за тем, чтобы у Вас не было пробелов в путях к файлам. Также обновите NodeJS, если давно не обновляли.
Установка займет некоторое время (примерно 5 минут).
C:/> npm init react-app gift-cards-gallery
C:/> cd gift-cards-gallery
C:/> npm start
Папка src у нас должна иметь следующий вид:
D:.
│ index.css
│ index.js
│
└───components
App.css
App.js
Card.css
Card.js
Далее приведу содержимое каждого файла:
./src/index.css
body {
margin: 0;
font-family: 'Segoe UI', sans-serif;
}
./src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './components/App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
./src/components/App.js
import React from 'react';
import './App.css';
import GiftCard from './Card'
function App() {
const data = [
{ imgSrc: 'https://source.unsplash.com/200x200/?gift', name: 'Gift 1', description: 'Some description', price: 22.95 },
{ imgSrc: 'https://source.unsplash.com/201x200/?gift', name: 'Gift 2', description: 'Some description', price: 19.35 },
{ imgSrc: 'https://source.unsplash.com/202x200/?gift', name: 'Gift 3', description: 'Some description', price: 20.75 },
{ imgSrc: 'https://source.unsplash.com/203x200/?gift', name: 'Gift 4', description: 'Some description', price: 10.75 },
{ imgSrc: 'https://source.unsplash.com/204x200/?gift', name: 'Gift 5', description: 'Some description', price: 11.75 },
{ imgSrc: 'https://source.unsplash.com/205x200/?gift', name: 'Gift 6', description: 'Some description', price: 9.75 },
{ imgSrc: 'https://source.unsplash.com/205x201/?gift', name: 'Gift 7', description: 'Some description', price: 9.75 },
{ imgSrc: 'https://source.unsplash.com/205x202/?gift', name: 'Gift 8', description: 'Some description', price: 9.75 },
{ imgSrc: 'https://source.unsplash.com/205x203/?gift', name: 'Gift 9', description: 'Some description', price: 9.75 },
];
return (
<div className="App">
<h1>Gift Cards Collection</h1>
{data.map((entry,index) =>
<GiftCard {...entry} key={index} />
)}
</div>
);
}
export default App;
./src/components/App.css
body {
background: #f0f0f0;
}
.App {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
gap: 10px;
}
h1 {
text-align: center;
grid-column: span 3;
}
@media screen and (max-width: 460px) {
.App {
display: block;
}
h1 {
font-size: 1.1em;
color: blue;
}
}
./src/components/Card.css
.GiftCard {
border: 1px solid #c7c4c4;
padding: 15px;
border-radius: 5px;
background: #fff;
margin: 5px;
text-align: center;
line-height: 1.5em;
animation: box-shadow 3s ease;
cursor: pointer;
}
.GiftCard:hover {
box-shadow: 10px 10px 5px #aaaaaa;
}
.gc-image {
width: 100%;
}
.gc-name {
font-weight: bold;
font-size: 1.2em;
}
.gc-description {
font-size: 1em;
}
.gc-price {
color: green;
font-weight: bold;
}
.gc-price::before {
content: '$';
}
./src/components/Card.js
import React from 'react'
import './Card.css'
const GiftCard = ({imgSrc, name, description, price}) => {
return (
<div className="GiftCard">
<img className="gc-image" src={imgSrc} alt={name} />
<div className="gc-name">{name}</div>
<div className="gc-description">{description}</div>
<div className="gc-price">{price}</div>
</div>
);
}
export default GiftCard;
После создания всех файлов как в примерах выше, Вы получите приложение похоже на картинку в начале статьи.
-
-
Михаил Русаков
Комментарии (0):
Для добавления комментариев надо войти в систему.
Если Вы ещё не зарегистрированы на сайте, то сначала зарегистрируйтесь.