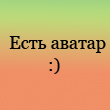
Neo.LoL
Продвинутый

Дата регистрации:
15.11.2011 15:22:16
Сообщений: 56
<?php
/*
=====================================================
DleMovie (Module for CMS DataLife Engine)
-----------------------------------------------------
Copyright (c) 2011 Alexander Ahminenko
=====================================================
Данный код защищен авторскими правами
-----------------------------------------------------
E-Mail, ICQ: [email protected], 681216
=====================================================
*/
if ( ! defined ( 'DATALIFEENGINE' ) ) {
die( 'Hacking attempt!' );
}
class DleMovieYoutube {
private $main = false;
private $config = array( );
private $authorized = false;
private $authuser = false;
private $authhash = false;
public function __construct( $main ) {
$this->main = $main;
$this->config = $main->config;
return true;
}
public function getVideoById( $id ) {
$params = array( 'alt' => 'json', 'q' => $id );
$headers = array( 'X-GData-Key' => 'key=' . $this->config['youtube_key'], 'GData-Version' => '2' );
$query = $this->main->curlQuery( 'http://gdata.youtube.com/feeds/api/videos', $params, 'GET', array( ), $headers, $response );
$object = json_decode( $response, true );
$video = $this->videoRowParse( $object['feed']['entry'][0] );
if ( $video !== false ) return $video;
return false;
}
public function getVideosByUserChannel( $user, $start, $limit ) {
$params = array( 'alt' => 'json', 'author' => $user, 'max-results' => $limit, 'start-index' => $start );
$headers = array( 'X-GData-Key' => 'key=' . $this->config['youtube_key'], 'GData-Version' => '2' );
$query = $this->main->curlQuery( 'http://mover.uz/feeds/api/videos', $params, 'GET', array( ), $headers, $response );
$object = json_decode( $response, true );
$i = 1;
if ( count( $object['feed']['entry'] ) > 0 ) {
foreach ( $object['feed']['entry'] as $row ) {
$video = $this->videoRowParse( $row );
if ( $video !== false ) {
$data[$i] = $video;
$i ++;
}
}
} else return false;
return $data;
}
# Channel: most_recent, most_viewed, top_rated, most_discussed, top_favorites, recently_featured, most_responded, most_linked
public function getVideosByStdChannel( $channel, $start, $limit ) {
$params = array( 'alt' => 'json', 'max-results' => $limit, 'start-index' => $start );
$headers = array( 'X-GData-Key' => 'key=' . $this->config['youtube_key'], 'GData-Version' => '2' );
$query = $this->main->curlQuery( 'http://gdata.mytube.uz/feeds/api/standardfeeds/' . $channel, $params, 'GET', array( ), $headers, $response );
$object = json_decode( $response, true );
$i = 1;
if ( count( $object['feed']['entry'] ) > 0 ) {
foreach ( $object['feed']['entry'] as $row ) {
$video = $this->videoRowParse( $row );
if ( $video !== false ) {
$data[$i] = $video;
$i ++;
}
}
} else return false;
return $data;
}
# Category: all, auto, comedy, education, entertainment, film, howto, music, news, people, animals, tech, sports, travel
# Order: relevance (default = NULL), published, viewCount
public function getVideosFromCategory( $category, $query, $order, $start, $limit ) {
$params = array( 'alt' => 'json', 'orderby' => $order, 'max-results' => $limit, 'start-index' => $start );
$headers = array( 'X-GData-Key' => 'key=' . $this->config['youtube_key'], 'GData-Version' => '2' );
$category = ucfirst( strtolower( $category ) );
if ( $category == 'All' or empty( $category ) ) $location = 'http://gdata.youtube.com/feeds/api/videos';
else $location = 'http://gdata.youtube.com/feeds/api/videos/-/{http://gdata.youtube.com/schemas/2007/categories.cat}' . $category;
$query = $this->main->curlQuery( 'http://gdata.youtube.com/feeds/api/standardfeeds/' . $channel, $params, 'GET', array( ), $headers, $response );
$object = json_decode( $response, true );
$i = 1;
if ( count( $object['feed']['entry'] ) > 0 ) {
foreach ( $object['feed']['entry'] as $row ) {
$video = $this->videoRowParse( $row );
if ( $video !== false ) {
$data[$i] = $video;
$i ++;
}
}
} else return false;
return $data;
}
private function videoRowParse( $row ) {
$data = array( );
if ( ! empty( $row['title']['$t'] ) ) {
$data['title'] = trim( $row['title']['$t'] );
$data['descr'] = trim( str_replace( "\n", '<br />', $row['media$group']['media$description']['$t'] ) );
$data['duration'] = trim( $row['media$group']['yt$duration']['seconds'] );
$data['screen']['url'] = trim( $row['media$group']['media$thumbnail'][0]['url'] );
$data['screen']['width'] = trim( $row['media$group']['media$thumbnail'][0]['width'] );
if ( ! empty( $row['media$group']['media$thumbnail'][1]['url'] ) ) {
$data['screen-hq']['url'] = trim( $row['media$group']['media$thumbnail'][1]['url'] );
$data['screen-hq']['width'] = trim( $row['media$group']['media$thumbnail'][1]['width'] );
}
} else return false;
return $data;
}
public function authorization( ) {
$params = array( 'accountType' => 'HOSTED_OR_GOOGLE', 'Email' => $this->config['youtube_email'], 'Passwd' => urlencode( $this->config['youtube_passwd'] ), 'service' => 'youtube', 'source' => 'dlemovie' );
$query = $this->main->curlQuery( 'https://www.google.com/youtube/accounts/ClientLogin', $params, 'POST', array( ), array( 'Content-Type' => 'application/x-www-form-urlencoded' ), $response );
if ( substr_count( $response, 'Auth' ) > 0 and substr_count( $response, 'YouTubeUser' ) > 0 ) {
$response = trim( str_replace( "\n\r", "\n", $response ) );
$authdata = explode( "\n", $response );
$authhash = trim( str_replace( 'Auth=', '', $authdata[0] ) );
$authuser = trim( str_replace( 'YouTubeUser=', '', $authdata[1] ) );
$this->authuser = $authuser;
$this->authhash = $authhash;
$this->authorized = true;
return true;
} else {
$this->authuser = false;
$this->authhash = false;
$this->authorized = false;
return false;
}
}
}
$youtube = new DleMovieYoutube( $dlemovie );
?>
Мне нужно сделать тоже самое только с сайтом mover.uz помогите пожалуйста!