Создание QR кода в C# WinForms с помощью библиотеки QRCoder
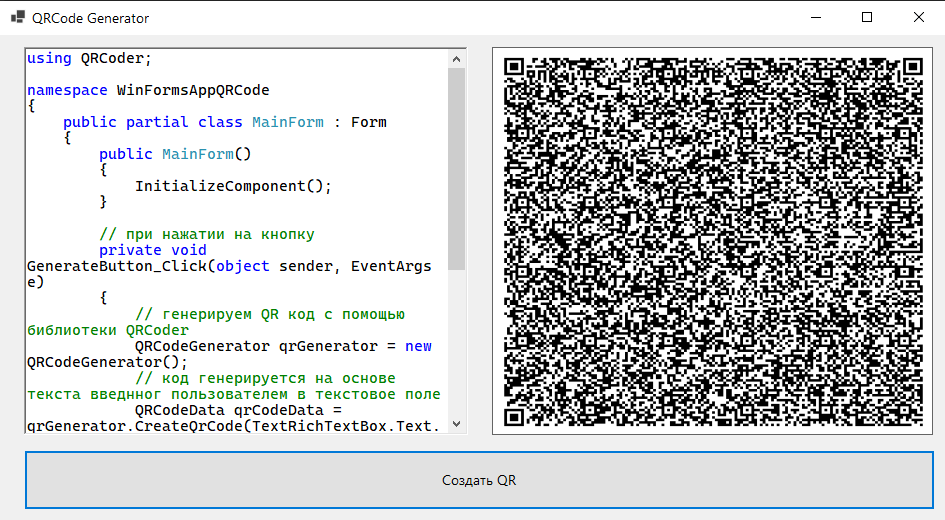
Доброго времени суток! QR-коды прочно вошли в нашу жизнь, как известно. В данном примере мы напишем программу для создания этого кода.
Итак, код:
Файл MainForm.Designer.cs
namespace WinFormsAppQRCode
{
partial class MainForm
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.ImagePictureBox = new System.Windows.Forms.PictureBox();
this.TextRichTextBox = new System.Windows.Forms.RichTextBox();
this.GenerateButton = new System.Windows.Forms.Button();
((System.ComponentModel.ISupportInitialize)(this.ImagePictureBox)).BeginInit();
this.SuspendLayout();
//
// ImagePictureBox
//
this.ImagePictureBox.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.ImagePictureBox.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle;
this.ImagePictureBox.Location = new System.Drawing.Point(492, 12);
this.ImagePictureBox.Name = "ImagePictureBox";
this.ImagePictureBox.Size = new System.Drawing.Size(441, 388);
this.ImagePictureBox.TabIndex = 0;
this.ImagePictureBox.TabStop = false;
//
// TextRichTextBox
//
this.TextRichTextBox.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.TextRichTextBox.Location = new System.Drawing.Point(24, 12);
this.TextRichTextBox.Name = "TextRichTextBox";
this.TextRichTextBox.Size = new System.Drawing.Size(444, 388);
this.TextRichTextBox.TabIndex = 1;
this.TextRichTextBox.Text = "";
//
// GenerateButton
//
this.GenerateButton.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.GenerateButton.Location = new System.Drawing.Point(24, 415);
this.GenerateButton.Name = "GenerateButton";
this.GenerateButton.Size = new System.Drawing.Size(911, 60);
this.GenerateButton.TabIndex = 2;
this.GenerateButton.Text = "Создать QR";
this.GenerateButton.UseVisualStyleBackColor = true;
this.GenerateButton.Click += new System.EventHandler(this.GenerateButton_Click);
//
// MainForm
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 19F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(945, 487);
this.Controls.Add(this.GenerateButton);
this.Controls.Add(this.TextRichTextBox);
this.Controls.Add(this.ImagePictureBox);
this.Name = "MainForm";
this.Text = "QRCode Generator";
((System.ComponentModel.ISupportInitialize)(this.ImagePictureBox)).EndInit();
this.ResumeLayout(false);
}
#endregion
private PictureBox ImagePictureBox;
private RichTextBox TextRichTextBox;
private Button GenerateButton;
}
}
Файл MainForm.cs
using QRCoder;
namespace WinFormsAppQRCode
{
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
}
// при нажатии на кнопку
private void GenerateButton_Click(object sender, EventArgs e)
{
// генерируем QR код с помощью библиотеки QRCoder
QRCodeGenerator qrGenerator = new QRCodeGenerator();
// код генерируется на основе текста введнног пользователем в текстовое поле
QRCodeData qrCodeData = qrGenerator.CreateQrCode(TextRichTextBox.Text.Trim(), QRCodeGenerator.ECCLevel.Q);
QRCode qrCode = new QRCode(qrCodeData);
// 5 - это размер 1-ого пикселя кода
Bitmap qrCodeImage = qrCode.GetGraphic(5);
// вставляем изображение в виджет, подгоняя размер QR кода к размеру родительского элемента ImagePictureBox
ImagePictureBox.Image = new Bitmap(qrCodeImage, ImagePictureBox.Width, ImagePictureBox.Height);
}
}
}
Program.cs
namespace WinFormsAppQRCode
{
internal static class Program
{
[STAThread]
static void Main()
{
ApplicationConfiguration.Initialize();
Application.Run(new MainForm());
}
}
}
Таким образом в данном пример мы создали простое приложение для создания QR-кодов в WinForms C#.
-
-
Михаил Русаков
Комментарии (0):
Для добавления комментариев надо войти в систему.
Если Вы ещё не зарегистрированы на сайте, то сначала зарегистрируйтесь.